useOrderLineItem
This hook is used to get the order line item data. Used in orderDetail page
.
Param | Type | Description | Example |
---|---|---|---|
title | string | title of the product | " Red Shoe" |
featureImg | string | feature image of the product | "https://cdn.shopify.com/url/to/image.jpg" |
variantTitle | string | variant title of the product | "Red 42" |
quantity | number | quantity of the product | "2" |
lineItemPrice | number | total price of line item of a single product after discount with currency | "Rs. 100" |
singleProductPrice | number | Actual price of the single product with mrp discount | "100" |
singleRegularPrice | number | Regular price of the single product without mrp discount | "100" |
singleProductPriceWithCurrency | string | Actual price with currency of the single product with mrp discount | "Rs. 100" |
singleRegularPriceWithCurrency | string | Regular price with currency of the single product without mrp discount npm | "Rs. 100" |
singleProductPriceDiscountWithCurrency | string | Total discount with currency of the single product with mrp discount and other discounts. | "Rs. 100" |
vendor | string | vendor of the product | "Nike" |
orderId | string | order id of the product | "1234567890" |
status | string | status of the product | "Delivered" |
openProduct | function | function to open product | openProduct() |
order | object | order object | order |
lineItem | object | line item of the product object | lineItem |
totalLineItemPriceWithCurrency | string | total price of line item of a product after discount multiplied by quantity | "Rs. 100" |
Example
const OrderLineItem = (props) => {
const {
title,
featureImg,
variantTitle,
quantity,
lineItemPrice,
singleProductPrice,
singleRegularPrice,
singleProductPriceWithCurrency,
singleRegularPriceWithCurrency,
singleProductPriceDiscountWithCurrency,
vendor,
orderId,
status,
openProduct,
order,
lineItem,
} = useOrderLineItem(props);
return (
<View>
<Text>{title} </Text>
</View>
);
};
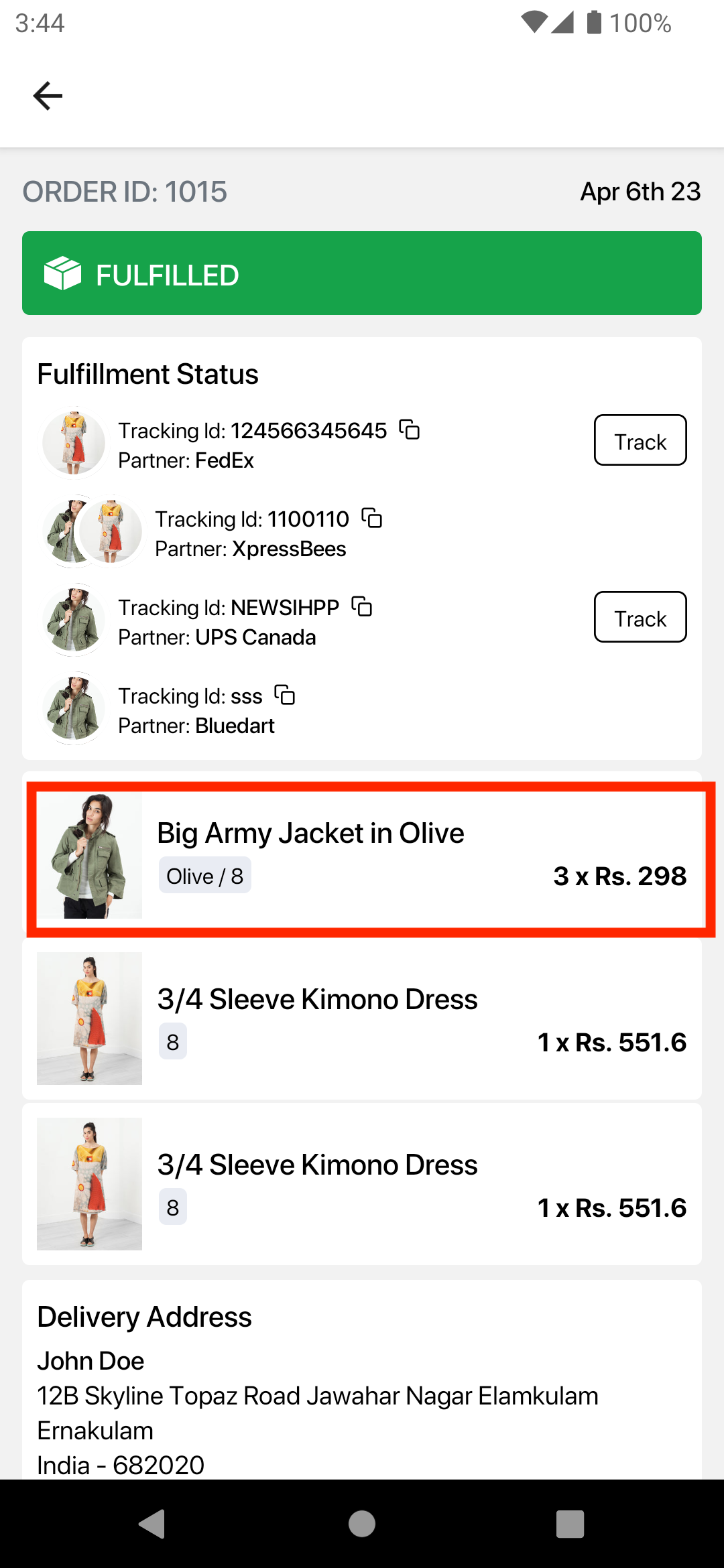