Customize Cart Card
The Cart Card is used to display the product details in the cart. It is used in the cart page.
Examples of customized Cart Card
The Cart Card can be customized using the theme. The following sections describe how to customize the Cart Card.
Steps to customize
- create a folder named
components
in thesrc
folder of the theme. - create a file named
CartCard.js
in thecomponents
orcomponents/cart
folder.
src/components/CartCard.js
import React from "react";
import {
View,
Text,
Image,
Pressable,
ActivityIndicator,
StyleSheet,
} from "react-native";
import Icon from "react-native-vector-icons/Feather";
import { useCartProduct } from "@appmaker-xyz/shopify";
const CartCard = (props) => {
const {
title,
imageUri,
variationText,
onSale,
onRemoveItem,
removeLoading,
lineItemSubTotalPriceWithCurrency,
variantLineItemRegularPriceWithCurrency,
} = useCartProduct(props);
return (
<View style={styles.container}>
<Image
source={{ uri: imageUri }}
resizeMode="contain"
style={styles.image}
/>
<View style={styles.contentContainer}>
<View style={styles.titleContainer}>
<Text numberOfLines={2}>{title}</Text>
<Pressable
style={styles.deleteIcon}
disabled={removeLoading}
onPress={onRemoveItem}
>
{removeLoading ? (
<ActivityIndicator size={16} color="#212121" />
) : (
<Icon name="trash-2" size={16} color="#212121" />
)}
</Pressable>
</View>
<View style={styles.rowBetweenContainer}>
<Text numberOfLines={2}>{variationText}</Text>
<View style={styles.priceContainer}>
{onSale ? (
<Text style={styles.regularPrice}>
{variantLineItemRegularPriceWithCurrency}
</Text>
) : null}
<Text>{lineItemSubTotalPriceWithCurrency}</Text>
</View>
</View>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
backgroundColor: "#fff",
flexDirection: "row",
borderBottomColor: "#EEEEEE",
borderBottomWidth: 1,
},
image: {
height: 120,
width: 100,
},
contentContainer: {
flex: 1,
justifyContent: "space-between",
padding: 12,
},
titleContainer: {
flexDirection: "row",
justifyContent: "space-between",
marginLeft: 6,
},
deleteIcon: {
backgroundColor: "#eee",
borderRadius: 16,
height: 28,
width: 28,
alignItems: "center",
justifyContent: "center",
},
rowBetweenContainer: {
flexDirection: "row",
alignItems: "center",
justifyContent: "space-between",
marginLeft: 6,
},
priceContainer: {
flexDirection: "row",
alignItems: "center",
},
regularPrice: {
marginRight: 4,
textDecorationLine: "line-through",
},
});
export default CartCard;
useCartProduct
is a custom hook that returns the product details from the cart. It is used to get the product details from the cart. For more information, see useCartProduct.
- create a folder named
blocks
in thesrc
folder of the theme. - create a file named
index.js
in theblocks
folder. - register the block in the
index.js
file as appmaker/cartCard.
src/blocks/index.js
import CollectionListItem from "../components/CollectionListItem";
import ProductData from "../components/ProductData";
import CartCard from "../components/CartCard";
const blocks = [
{
name: "appmaker/product-grid-item",
View: CollectionListItem,
},
{
name: "appmaker/shopify-product-data",
View: ProductData,
},
{
name: "appmaker/cartCard",
View: CartCard,
},
];
export { blocks };
- Run the app and check the changes.
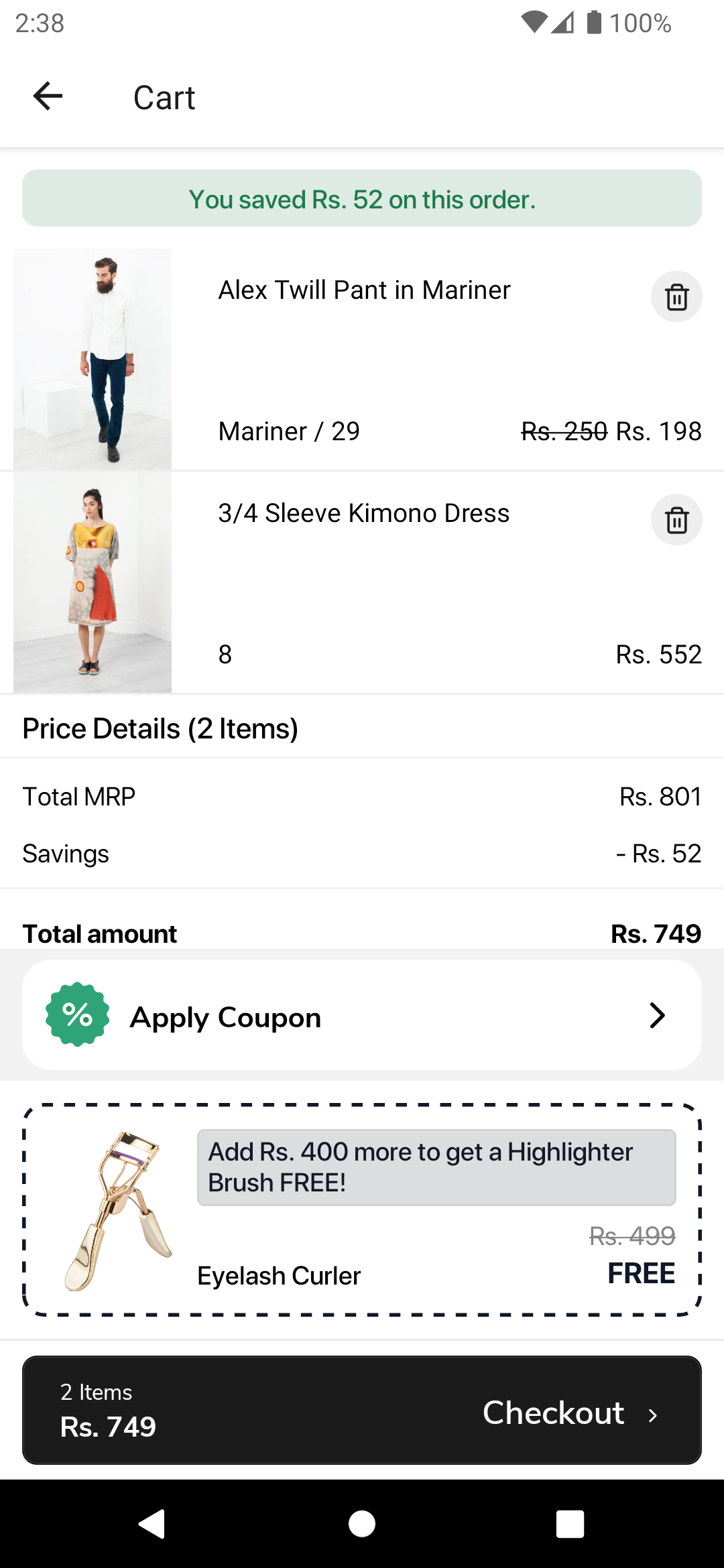
info
Detailed information about customizing the Cart Card can be found in the Customizing Cart Card section.
Sample Design
The following file contain a sample design for the Cart page. You can use this design as a reference to customize the Cart Card. Sample Design