Add custom blocks in product list Page
This guide will help you add custom blocks in product list view. We can render custom blocks inside listview of products.
Use case
- Display a banner after every 10 products in the listview.
- Display a quick filter by size when customer reaches 30 products in the listview.
- Display a countdown timer when customer reaches 50 products in the listview.
Hook
If you are handling the products in product list page, you can use the same hook to inject the custom blocks. The hook is shopify-custom-products-response
.
Steps
First we need to modify the product data and inject the custom blocks in the response.
appmaker.addFilter(
"shopify-custom-products-response",
"appmaker-custom-blocks",
(_currentResponse, params, dependencies = {}) => {
const collectionId = shopifyIdHelper(params?.collectionId, true);
const products = getProducts({ collectionId }); // let us assume this function returns the products
// The 'surface' parameter indicates which list is being called. For example, if the list is from the collection, its value will be 'collection-page'.
const surface = params.surface;
const bannerBlock = {
___appmakercustomblock: {
enabled: true,
block: {
name: "appmaker/image",
attributes: {
title: "Banner",
description: "This is a banner",
url: "https://cdn.shopify.com/s/files/1/0533/2089/files/placeholder-images-image_large.png?format=webp&v=1530129081", // image url
width: 1440, // image width
height: 593, // image height
appmakerAction: {
action: "OPEN_COLLECTION", // action type
params: {
collectionHandle: "all", // collection handle
},
},
},
},
},
};
// productsList
// inject the banner block after every 10 products
// products is the list of products
const productsList = products.flatMap((product, index) => {
if (index % 10 === 0) {
return [product, bannerBlock];
}
return product;
});
return {
data: {
data: {
products: {
edges: productsList,
},
},
},
};
}
);
You can see the above code, we are injecting the banner block after every 10 products.
Output
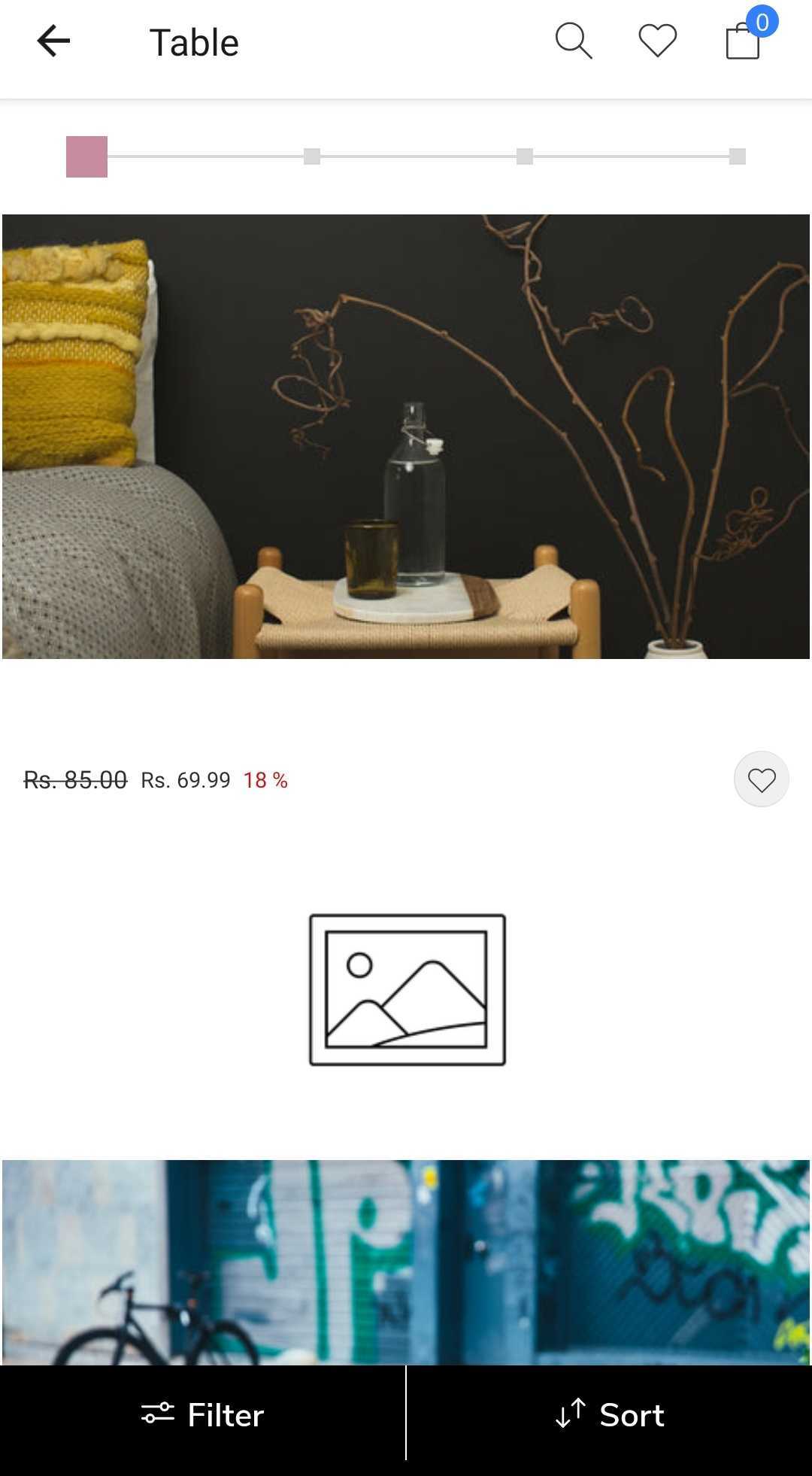
Similarly, you can inject the custom blocks in the product list page based on your use case.
const block = {
___appmakercustomblock: {
enabled: true,
block: {
name: "appmaker/count-down-timer",
attributes: {
title: "Countdown Timer",
description: "This is a countdown timer",
date: "2023-03-29T12:43:15.708Z",
appmakerAction: {
action: "OPEN_COLLECTION", // action type
params: {
collectionHandle: "all", // collection handle
},
},
},
},
},
};
Output
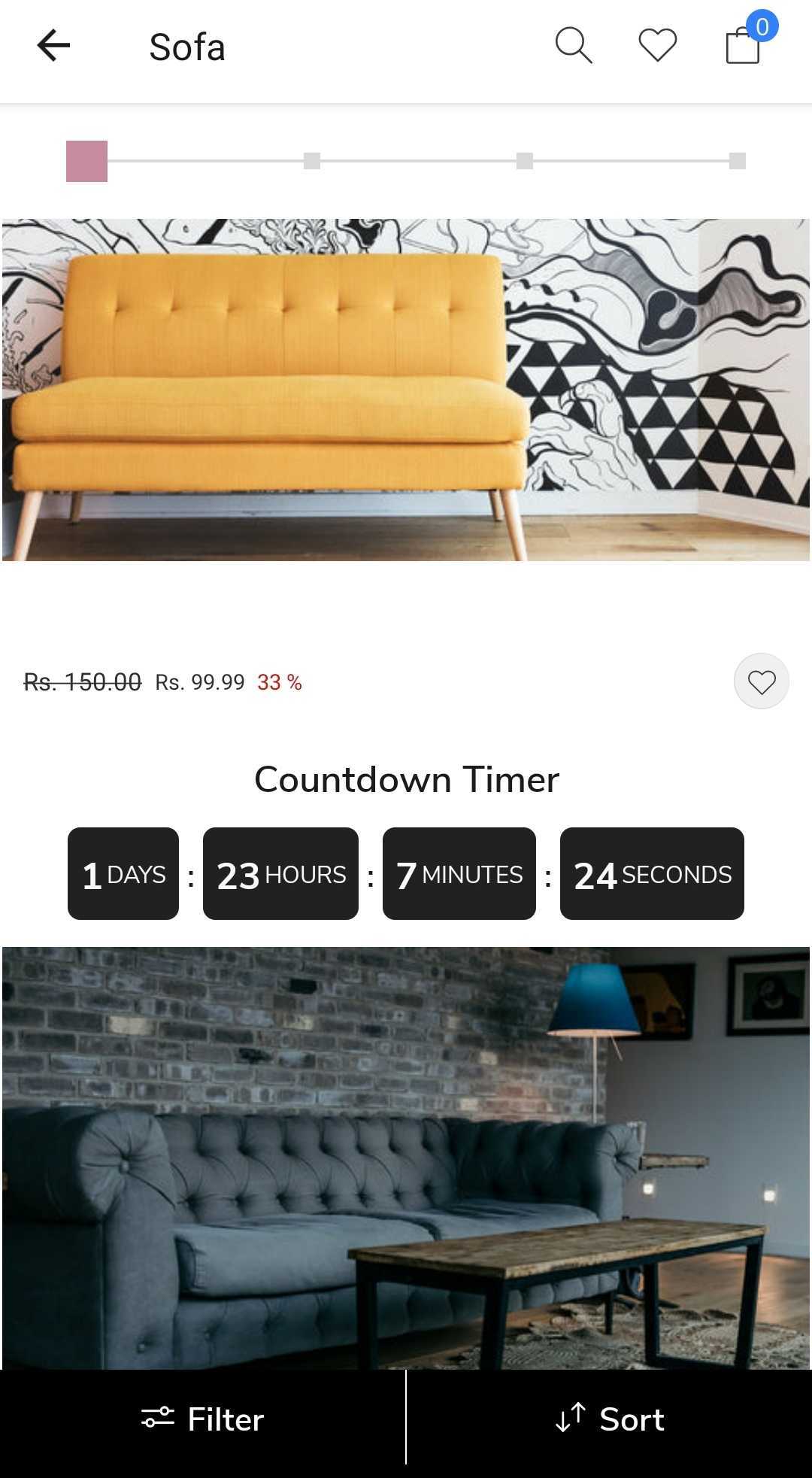