How to add metafields to product
Introduction
In this guide, we will show you how to add meta fields to the product, so that you can use them in the app. Let's say you want to add a related products list to the product page. You can add a metafield to the product and then use it in the app.
Let's divide this guide into two parts:
- Adding metafields to the product in the shopify admin
- How to use the metafields in the app
Adding metafields to the product in the shopify admin
- Go to the Shopify admin and click on settings.
- Go to custom data and click on 'products'.
- Click on 'Add definition'.
- Now create a new metafield with the following details:
- Name:
related_products
// sample name - Namespace and key:
custom.products_list
- Click Select content type and select 'product'
- To allow a list of products in your metafield, select list of products.
- select storefront from the access option on right side
- click on save.
- Name:
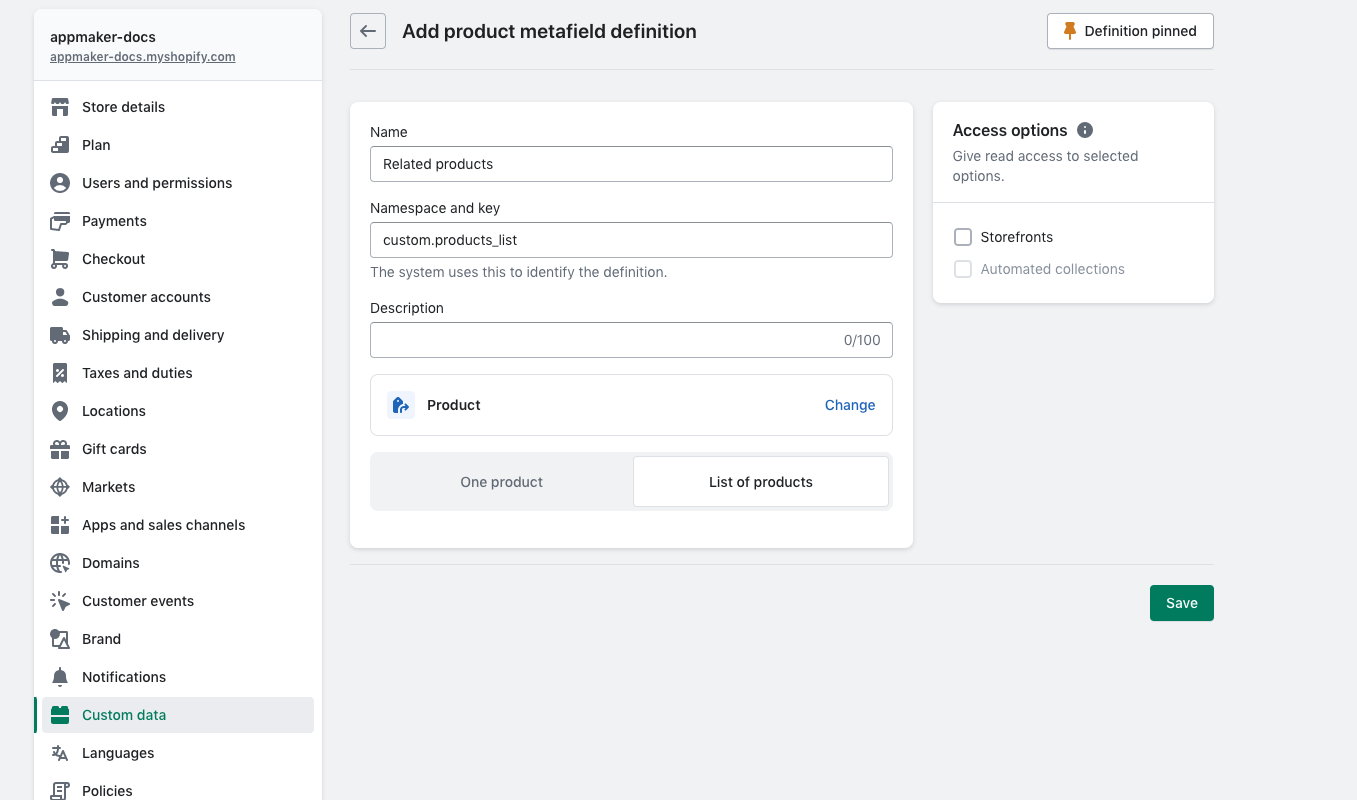
- Now go to the single product page and navigate to the botton of the page. You can see the metafields section there.
- In
related products
metafield, select the products you want to show in the related products list. - Click on save.
Now you are done with adding metafields to the product in the shopify admin.
How to use the metafields in the app
- Create a file functions.js in the packages/your-package-name/ directory.
- Add the following code to the functions.js file:
import { fieldsHelper } from "@appmaker-xyz/shopify";
export function addProductCollection() {
fieldsHelper.addFieldsToProduct({
fields: {
// add any fields in the required format
custom_products_list: {
__aliasFor: 'metafield',
__args: {
key: 'products_list',
namespace: 'custom',
},
value: true,
},
},
});
}
In the above code key is the key of the metafield and namespace is the namespace of the metafield which you have added in the product from shopify admin. Here the key is "products_list" and namespace is "custom". so the key for the field is set in the format "custom_products_list".
- Add the following code to the index.js file:
import { addProductCollection } from './functions';
// call this function in the activate function
addProductCollection();
- After doing this you will be able to get the custom field in the product query.
- Go to your pages/productDetail.js and create a new custom related product list block like below:
{
name: 'my-theme/custom-related-products',
clientId: 'custom-related-products',
attributes: {
productIds: '{{blockItem.node.custom_products_list}}'
},
}
- then register the block in the blocks/index.js file:
import CustomRelatedProducts from '../components/customRelatedProducts.js';
// register the block
{
name: 'my-theme/custom-related-products',
View: customRelatedProducts,
}
- Then create a customRelatedProducts.js file in the components directory and add the following code:
import React, { useEffect, useState } from 'react';
import { View, Text } from 'react-native';
import { runDataSource } from '@appmaker-xyz/core';
async function loadProducts(ids) {
const dataSource = {
attributes: {},
source: 'shopify',
};
const [response] = await runDataSource(
{
dataSource,
},
{
methodName: 'products',
params: {
ids, // product ids, eg: ['gid://shopify/Product/1234567890', 'gid://shopify/Product/0987654321']
},
},
);
const products = response.data.data.nodes;
}
export default function CustomRelatedProducts({ attributes }) {
const [products, setProducts] = useState([]);
const productIds = attributes.productIds;
useEffect(() => {
const ids = JSON.parse(productIds.value);
loadProducts(ids).then((products) => {
setProducts(products);
});
}, [productIds]);
return (
<View>
{products.map((product) => (
<Text>{product.title}</Text>
))}
</View>
);
}
or if you want to show the products as a scrollable list, you can use the following code:
import React, { useEffect, useState } from "react";
import { View, Text } from "react-native";
export default function CustomRelatedProducts({
attributes,
BlockItem,
onAction,
currentAction,
BlocksView,
innerBlocks,
blockData,
}) {
const productIds = attributes?.productIds?.value ? JSON.parse(attributes.productIds.value): null;
const finalAttributes = appmaker.applyFilters(
'shopify-product-scroller-attributes',
{
horizontal: true,
dataSource: {
source: 'shopify',
attributes: {
mapping: {
items: 'data.data.products.edges',
},
methodName: 'products',
params: {
ids: productIds,
productsLimit: 10,
},
},
repeatable: 'Yes',
repeatItem: 'DataSource',
},
appmakerAction: {
params: {
pageData: '{{ blockItem }}',
},
action: 'OPEN_PRODUCT_DETAIL',
},
},
);
if(!productIds) {
return null;
}
return (
<BlockItem
BlockItem={BlockItem}
BlocksView={BlocksView}
currentAction={currentAction}
onAction={onAction}
block={{
name: "appmaker/product-grid-item",
innerBlocks,
clientId: "product-list",
isValid: true,
attributes: finalAttributes,
}}
/>
);
}